.NET 8.0 の Windowsフォームアプリで、Dapper + SQLite NuGetパッケージを使い、SQLiteデータベースに CRUD処理するサンプルを作成しました。ORマッピングの主流は Dapper。
ソースコードは GitHub で公開しています。
データベース
SQLiteの管理ツールは DB Browser for SQLite を使用。
使用したバージョンは 2024/10/29時点で最新の v3.13.1。
https://sqlitebrowser.org/blog/version-3-13-1-released/
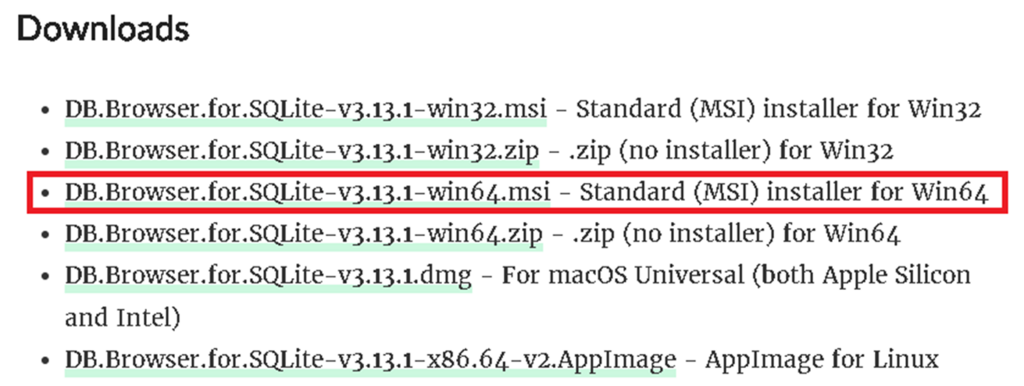
DB Browser for SQLite で SQLiteのデータベースファイルを作成。
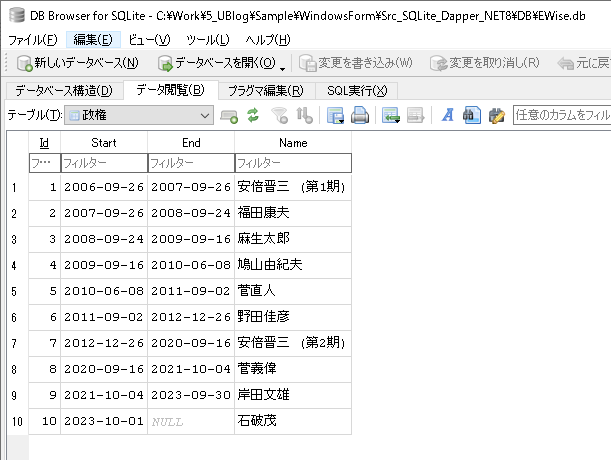
テーブル定義。
1 2 3 4 5 6 7 8 9 |
CREATE TABLE "政権" ( "Id" INTEGER, "Start" TEXT, "End" TEXT, "Name" TEXT, PRIMARY KEY("Id") ) |
※SQliteのデータベースファイルをパスワードで暗号化する機能は System.Data.SQLiteが 1.0.114 以降のバージョンでは使えないらしい。
c# – ファイルまたはアセンブリ ‘System.Data.SQLite.SEE.License、バージョン =1.0.117.0 – スタック オーバーフローを読み込めませんでした
SQLiteのパスワード有りデータベースファイルへ接続するテストツール
ソースコード構成
今回使った Visual Studio プロジェクト テンプレートは、Visual Studio 2022 + .NET 8 + Windowsフォームアプリ。
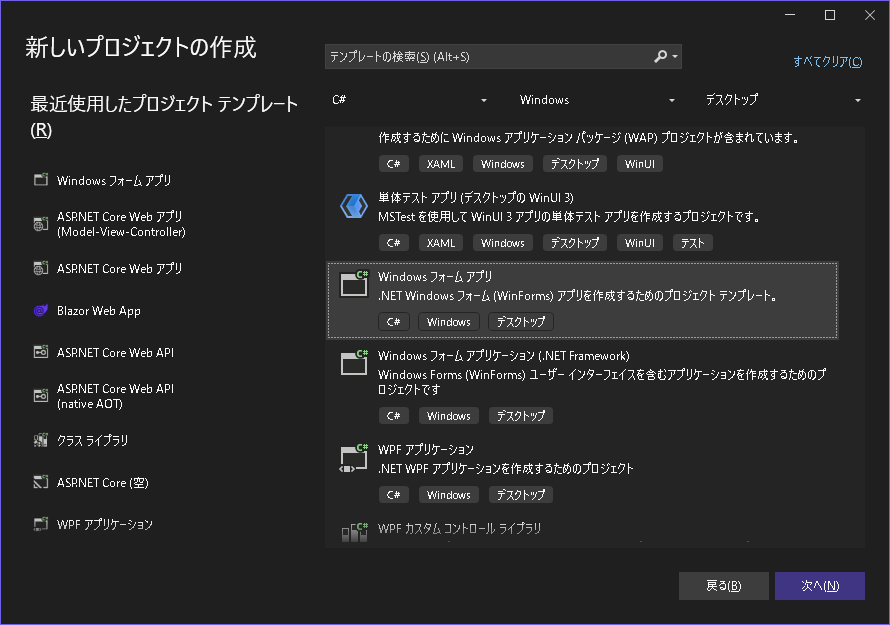
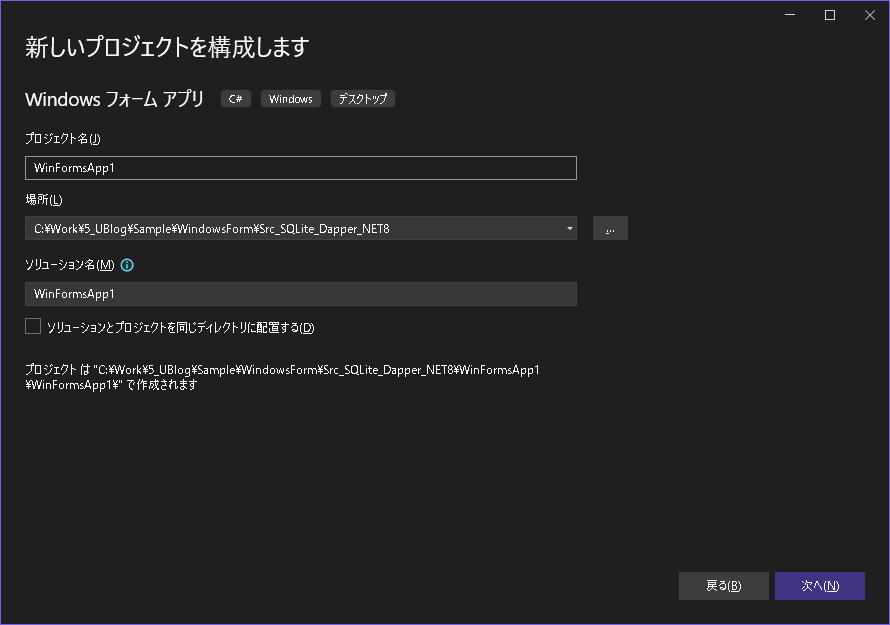
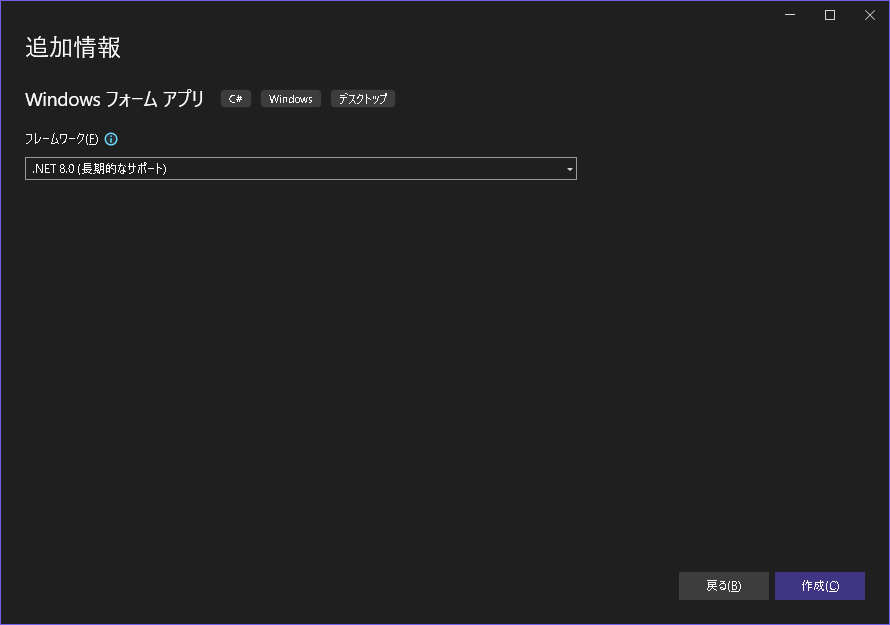
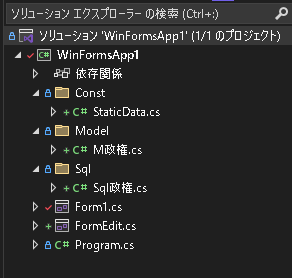
NuGet パッケージ インストール
System.Data.SQLiteパッケージをインストール
使用したバージョンは 2024/10/29時点で最新の v1.0.119。
1 2 3 |
> Install-Package System.Data.SQLite |
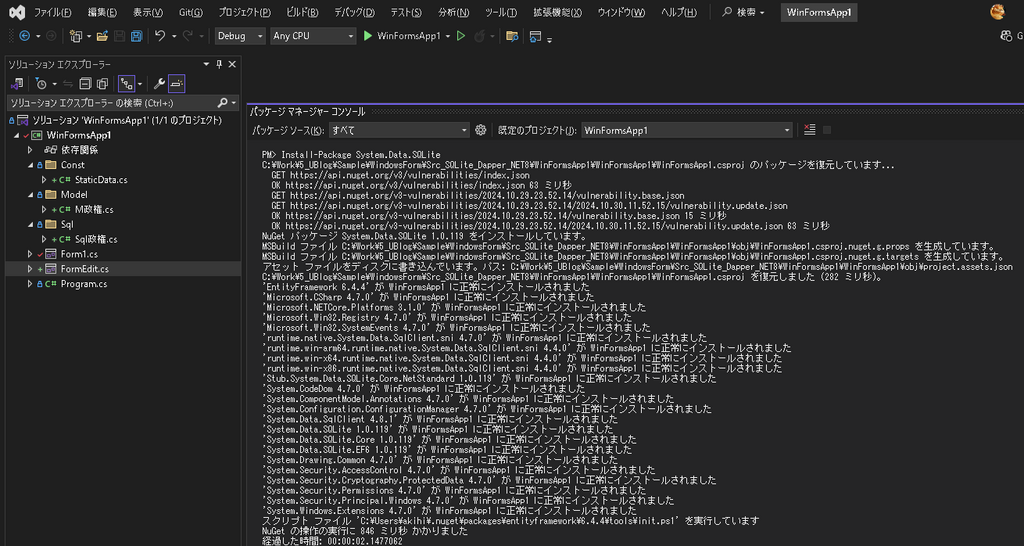
Dapperパッケージをインストール
使用したバージョンは 2024/10/29時点で最新の v2.1.35。
1 2 3 |
> Install-Package Dapper |
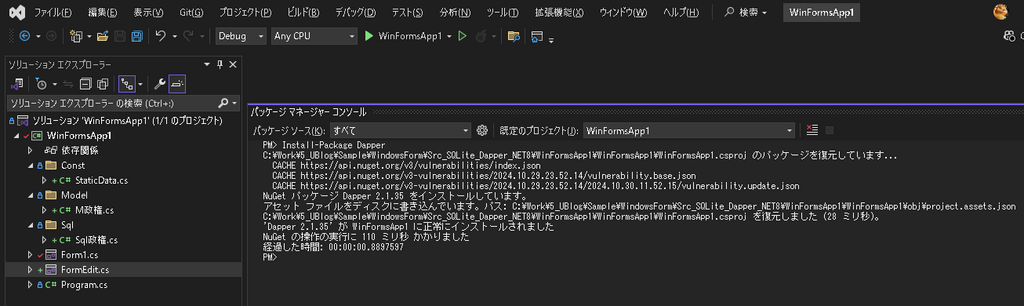
ソースコード変更内容を解説
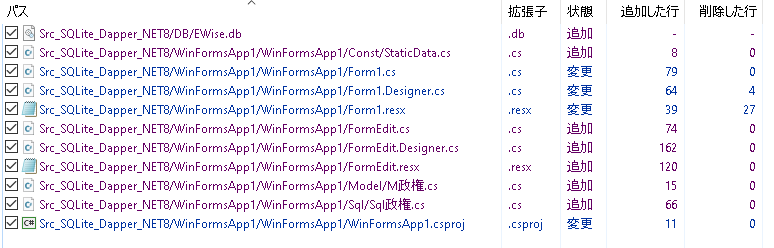
/DB/EWise.db
DB Browser for SQLiteで作成した SQLiteのデータベースファイル。
/WinFormsApp1/WinFormsApp1/Const/StaticData.cs
SQLiteのデータベースファイルに接続するコネクションストリングを追加。

1 2 3 4 5 6 7 8 9 |
namespace Maintenance.Common { public static class StaticData { public static string ConnectionString = @"Data Source=..\..\..\..\..\DB\EWise.db;"; } } |
/WinFormsApp1/WinFormsApp1/Form1.cs
アプリ起動時に表示されるメイン画面。
Form1_Load()イベントハンドラで SQLiteデータベースのデータを DataGridViewに表示。
btn追加_Click()イベントハンドラで編集画面を表示。
dataGridView1_CellContentClick()イベントハンドラで対象行の「編集」「削除」ボタンを処理。
Get政権()メソッドで SQLiteデータを DataGridViewに表示する処理を共通化。
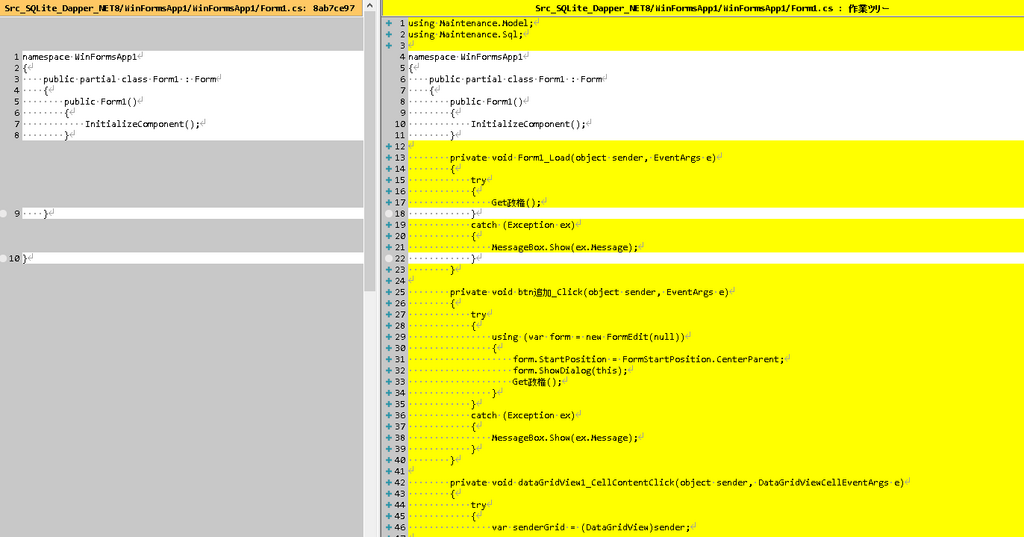
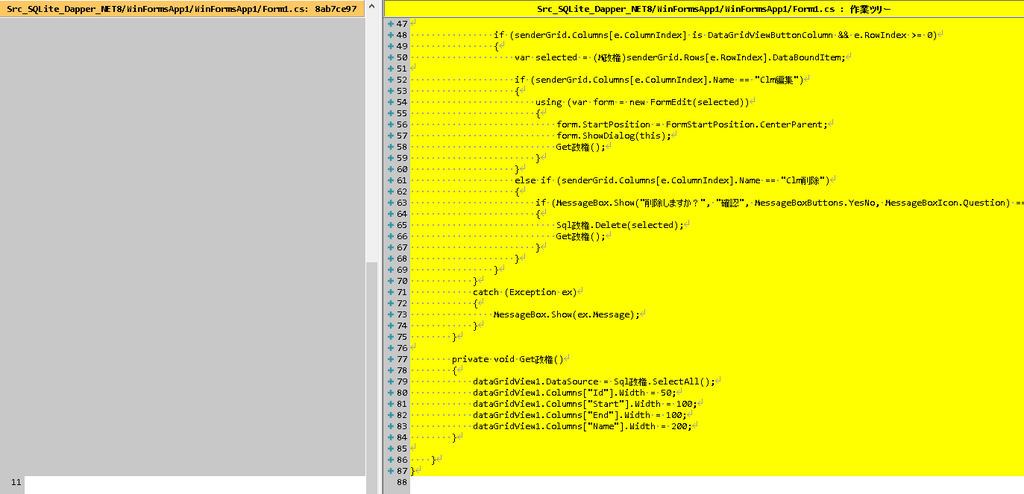
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
using Maintenance.Model; using Maintenance.Sql; namespace WinFormsApp1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { try { Get政権(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btn追加_Click(object sender, EventArgs e) { try { using (var form = new FormEdit(null)) { form.StartPosition = FormStartPosition.CenterParent; form.ShowDialog(this); Get政権(); } } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e) { try { var senderGrid = (DataGridView)sender; if (senderGrid.Columns[e.ColumnIndex] is DataGridViewButtonColumn && e.RowIndex >= 0) { var selected = (M政権)senderGrid.Rows[e.RowIndex].DataBoundItem; if (senderGrid.Columns[e.ColumnIndex].Name == "Clm編集") { using (var form = new FormEdit(selected)) { form.StartPosition = FormStartPosition.CenterParent; form.ShowDialog(this); Get政権(); } } else if (senderGrid.Columns[e.ColumnIndex].Name == "Clm削除") { if (MessageBox.Show("削除しますか?", "確認", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes) { Sql政権.Delete(selected); Get政権(); } } } } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void Get政権() { dataGridView1.DataSource = Sql政権.SelectAll(); dataGridView1.Columns["Id"].Width = 50; dataGridView1.Columns["Start"].Width = 100; dataGridView1.Columns["End"].Width = 100; dataGridView1.Columns["Name"].Width = 200; } } } |
/WinFormsApp1/WinFormsApp1/Form1.Designer.cs /WinFormsApp1/WinFormsApp1/Form1.resx
初期表示画面のレイアウトはこんな感じ。
アプリ起動時に表示されるメイン画面。
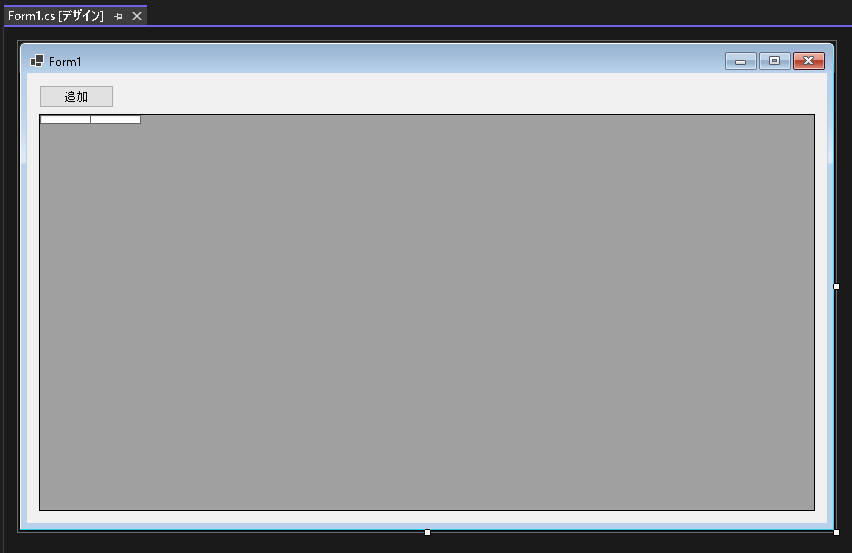
/WinFormsApp1/WinFormsApp1/FormEdit.cs
初期表示画面で「追加」「編集」ボタンをクリックした際に表示するデータ編集画面。
FormEdit()コンストラクタで 初期表示画面のDataGridViewの行データを受け取る。
FormEdit_Load()イベントハンドラで DataGridViewの行データを各テキストボックスに展開。
btn登録_Click()イベントハンドラで 入力された値を SQLiteデータベースへ反映。
btnキャンセル_Click()イベントハンドラは データ編集画面を閉じるだけ。
「return sqliteConnection.Query<M政権>(query).AsList();」で SQLiteデータベースから取得した M政権モデルクラスのデータを DataGridViewへ展開し、
「var selected = (M政権)senderGrid.Rows[e.RowIndex].DataBoundItem;」で DataGridViewのデータを M政権モデルクラスへ戻す流れがとてもシンプルになっている。
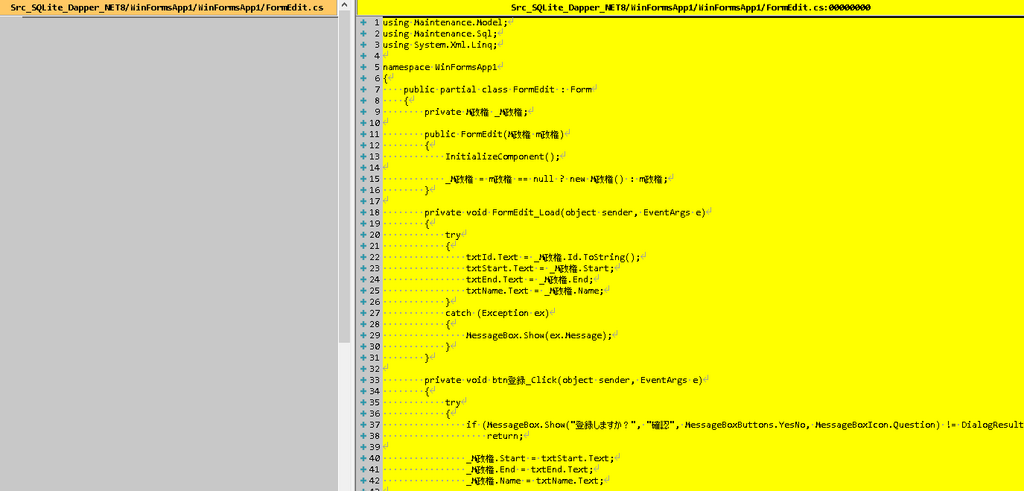
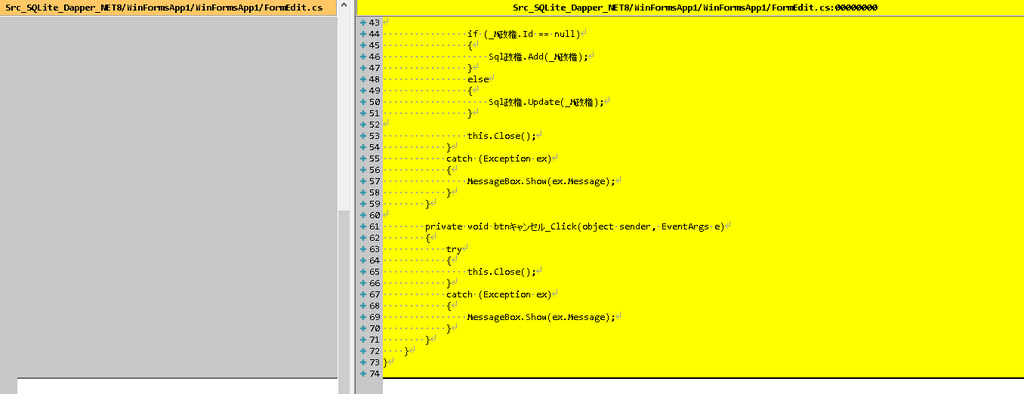
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
using Maintenance.Model; using Maintenance.Sql; using System.Xml.Linq; namespace WinFormsApp1 { public partial class FormEdit : Form { private M政権 _M政権; public FormEdit(M政権 m政権) { InitializeComponent(); _M政権 = m政権 == null ? new M政権() : m政権; } private void FormEdit_Load(object sender, EventArgs e) { try { txtId.Text = _M政権.Id.ToString(); txtStart.Text = _M政権.Start; txtEnd.Text = _M政権.End; txtName.Text = _M政権.Name; } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btn登録_Click(object sender, EventArgs e) { try { if (MessageBox.Show("登録しますか?", "確認", MessageBoxButtons.YesNo, MessageBoxIcon.Question) != DialogResult.Yes) return; _M政権.Start = txtStart.Text; _M政権.End = txtEnd.Text; _M政権.Name = txtName.Text; if (_M政権.Id == null) { Sql政権.Add(_M政権); } else { Sql政権.Update(_M政権); } this.Close(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btnキャンセル_Click(object sender, EventArgs e) { try { this.Close(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } } } |
/WinFormsApp1/WinFormsApp1/FormEdit.Designer.cs/WinFormsApp1/WinFormsApp1/FormEdit.resx
データ編集画面のレイアウトはこんな感じ。
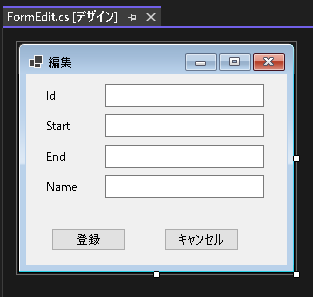
/WinFormsApp1/WinFormsApp1/Model/M政権.cs
SQLiteデータベースの 政権テーブルに対応する M政権モデルクラスを追加。
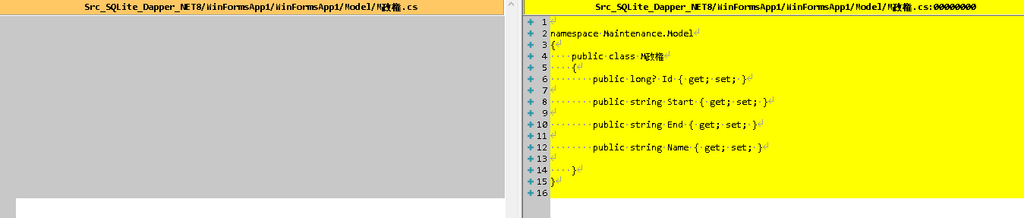
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
namespace Maintenance.Model { public class M政権 { public long? Id { get; set; } public string Start { get; set; } public string End { get; set; } public string Name { get; set; } } } |
/WinFormsApp1/WinFormsApp1/Sql/Sql政権.cs
SQLiteデータベースの 政権テーブルに対する CRUD処理を追加。
Dapperを使っていることで、SQLとデータの紐づけ、Selectしたデータを M政権モデルクラスへ変換する処理、M政権モデルクラスを Insert/Updateする処理がとてもシンプルになっている。
データベース処理に SQLを使わない開発者は無能。
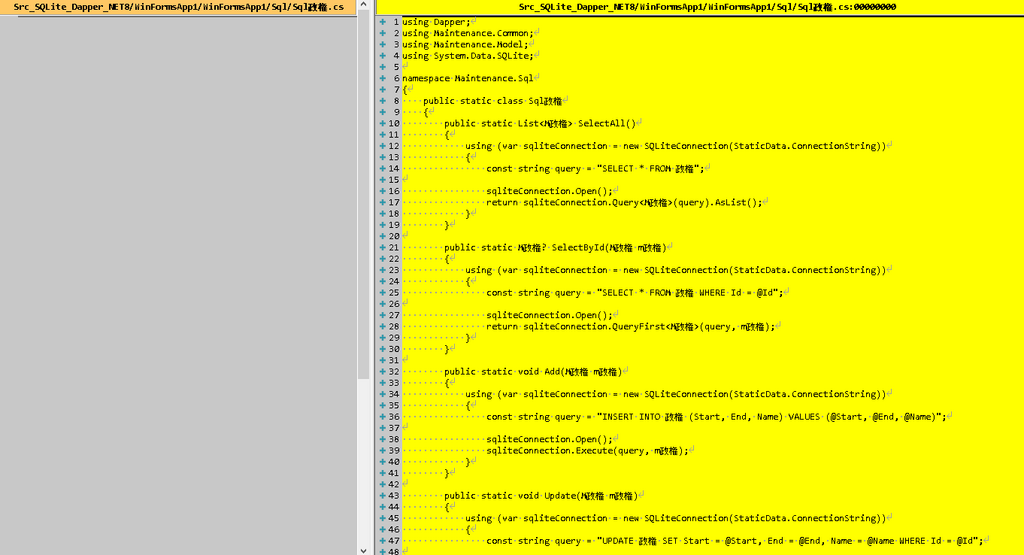
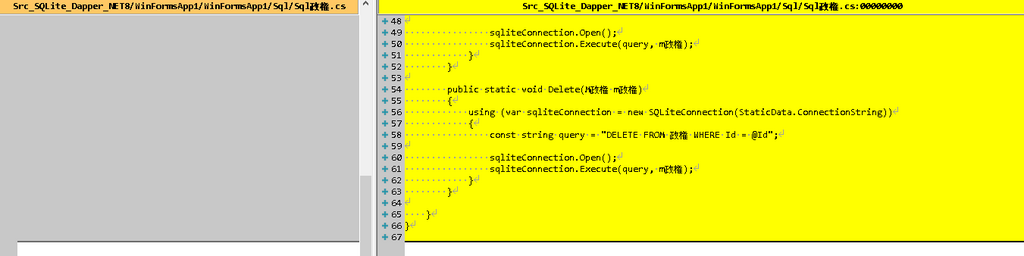
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
using Dapper; using Maintenance.Common; using Maintenance.Model; using System.Data.SQLite; namespace Maintenance.Sql { public static class Sql政権 { public static List<M政権> SelectAll() { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "SELECT * FROM 政権"; sqliteConnection.Open(); return sqliteConnection.Query<M政権>(query).AsList(); } } public static M政権? SelectById(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "SELECT * FROM 政権 WHERE Id = @Id"; sqliteConnection.Open(); return sqliteConnection.QueryFirst<M政権>(query, m政権); } } public static void Add(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "INSERT INTO 政権 (Start, End, Name) VALUES (@Start, @End, @Name)"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権); } } public static void Update(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "UPDATE 政権 SET Start = @Start, End = @End, Name = @Name WHERE Id = @Id"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権); } } public static void Delete(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "DELETE FROM 政権 WHERE Id = @Id"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権); } } } } |
/WinFormsApp1/WinFormsApp1/WinFormsApp1.csproj
インストールした NuGetパッケージや、追加したコンポーネントが、追加されている。
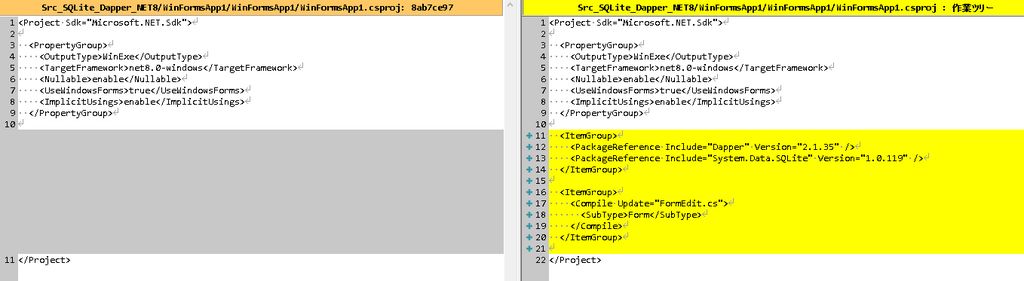
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <OutputType>WinExe</OutputType> <TargetFramework>net8.0-windows</TargetFramework> <Nullable>enable</Nullable> <UseWindowsForms>true</UseWindowsForms> <ImplicitUsings>enable</ImplicitUsings> </PropertyGroup> <ItemGroup> <PackageReference Include="Dapper" Version="2.1.35" /> <PackageReference Include="System.Data.SQLite" Version="1.0.119" /> </ItemGroup> <ItemGroup> <Compile Update="FormEdit.cs"> <SubType>Form</SubType> </Compile> </ItemGroup> </Project> |
コメント