10年くらい前、「世界中の Web API に自由に接続し、様々なデータを簡単に収集できる時代が来た」と騒がれていた時期がありましたが、最近、会社情報を収集する必要が出て、世界中のWeb API を利用する為のプラットフォームになっている RapidAPI を使ってみたら、そんな時代が来ていることを実感しました。
自分が使ったのは、 Rakuten RapidAPI の中にある、Yahoo Finance API。
アジア向けの RapidAPI サービスは、楽天が請け負っているらしい。
Yahoo Finance API の概要は、Yahoo Finance API – The Complete Guide に記載されている。
料金プランが明瞭。どのAPIも料金プランのレイアウトが同じなのは、混乱しなくて助かる。
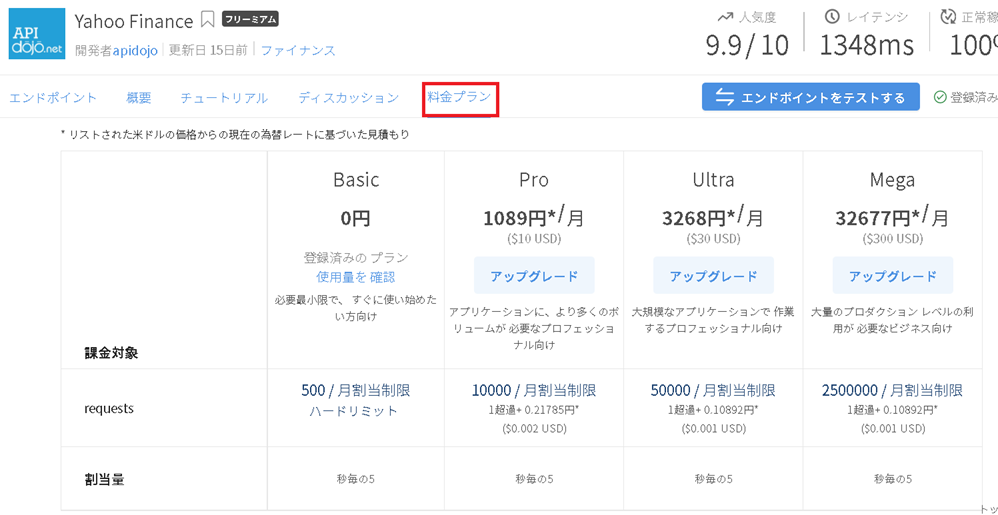
Yahoo Finance API は、アップルのような米国会社情報も収集できる。
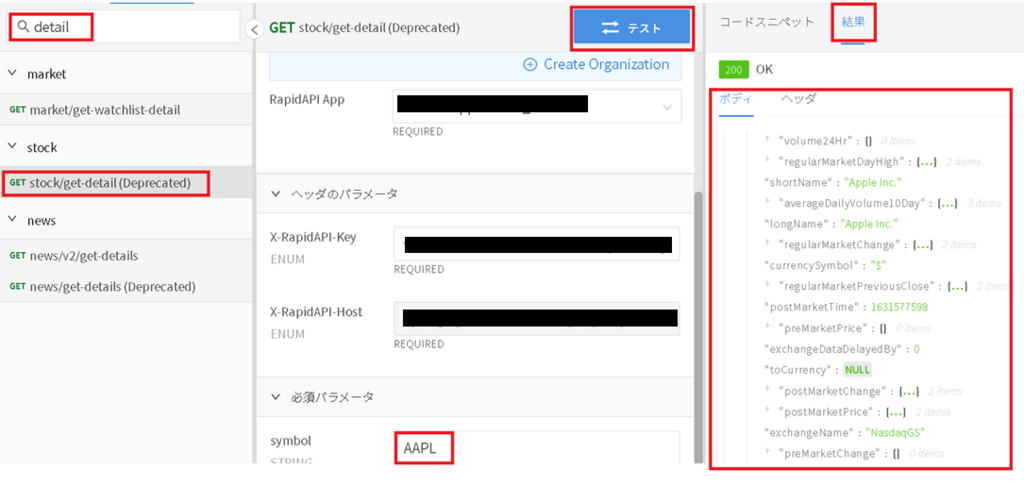
C#で収集する場合のソースコードも出力してくれる。
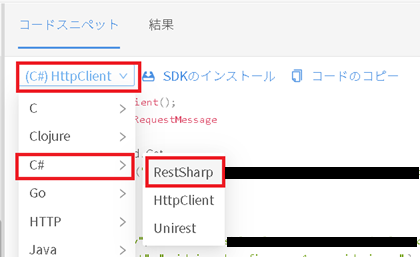
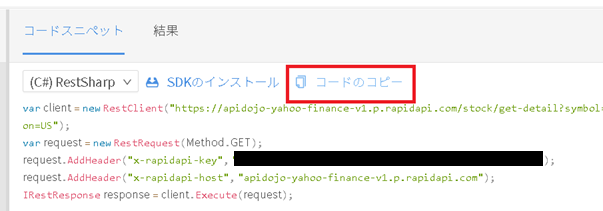
RestSharp形式の C#ソースコード実行には、 RestSharp Nugetパッケージのインストールが必要。
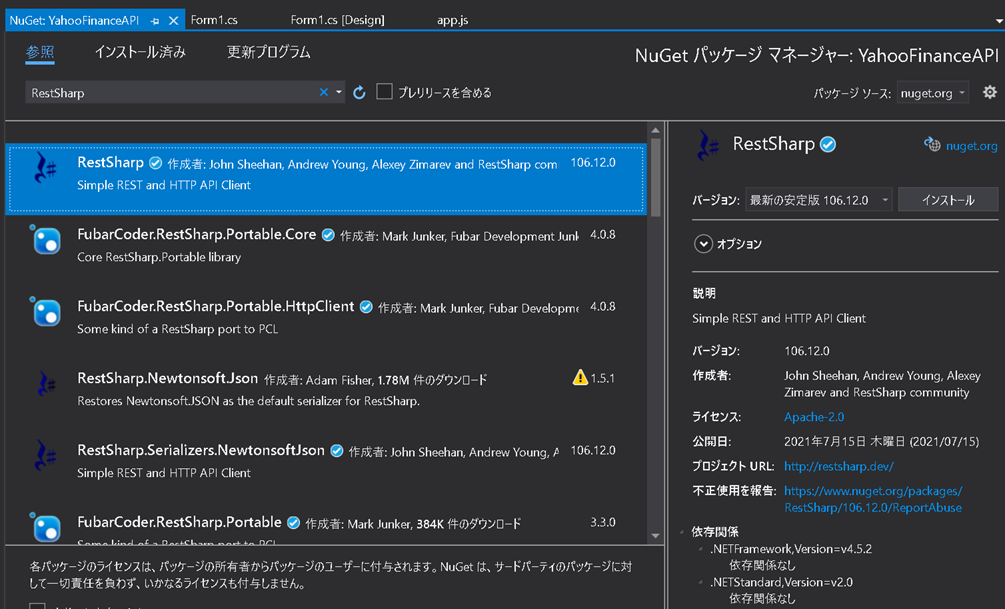
後は、Visual Studio のプロジェクトにソースコードを貼り付けて、実行するだけ。
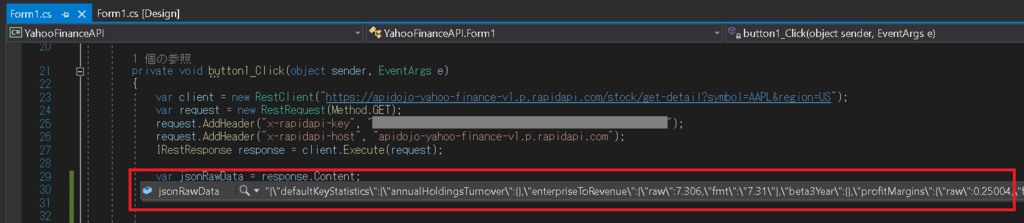
保存したJsonデータは、VisualStudioのドキュメントフォーマットを行うと見易くなる。
保存
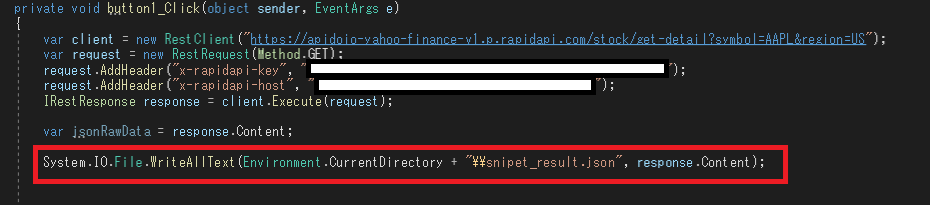
before

ドキュメントフォーマット実行
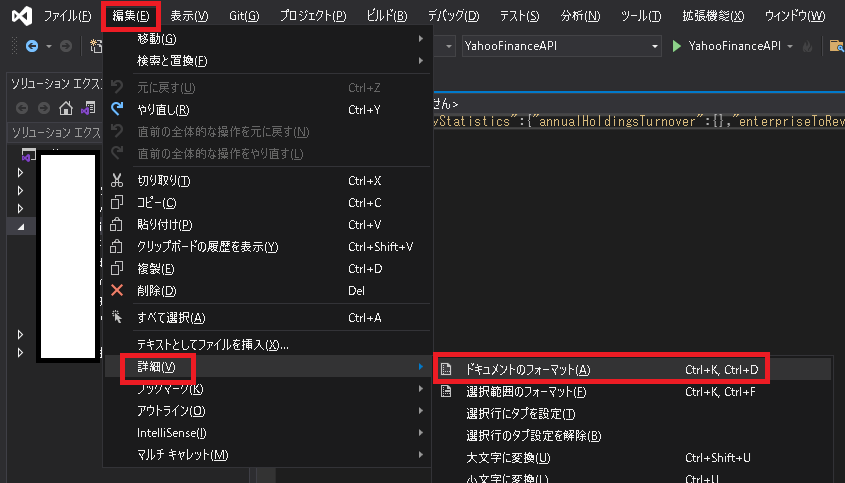
After
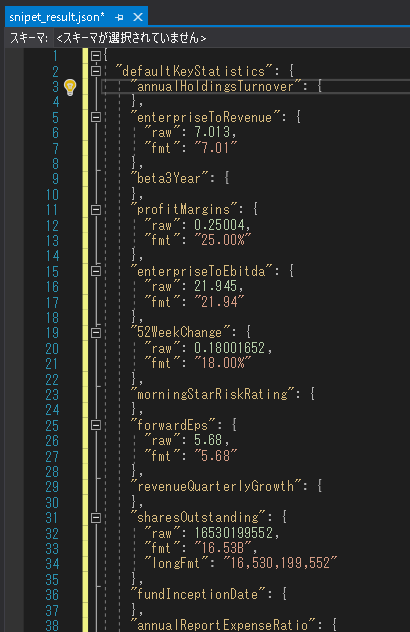
保存したJsonデータに対応するClassを作成する際は、「JSONをクラスとして貼り付ける」機能を使用すると便利。JSONデータのフォーマットに対応したデータクラスを自動生成してくれる。
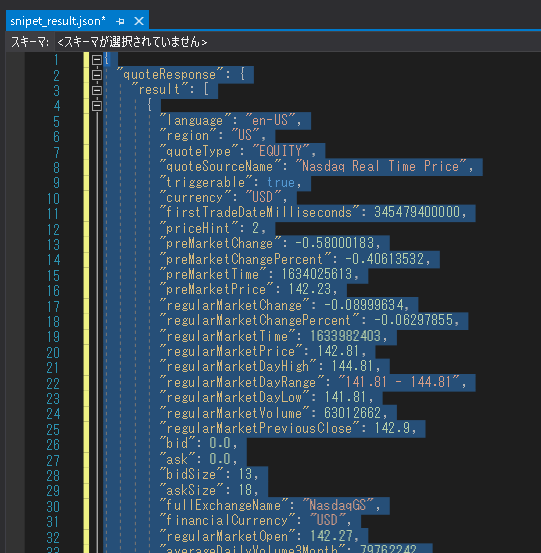
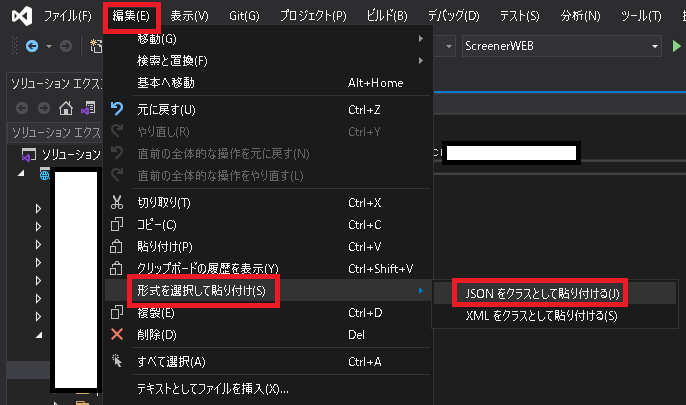
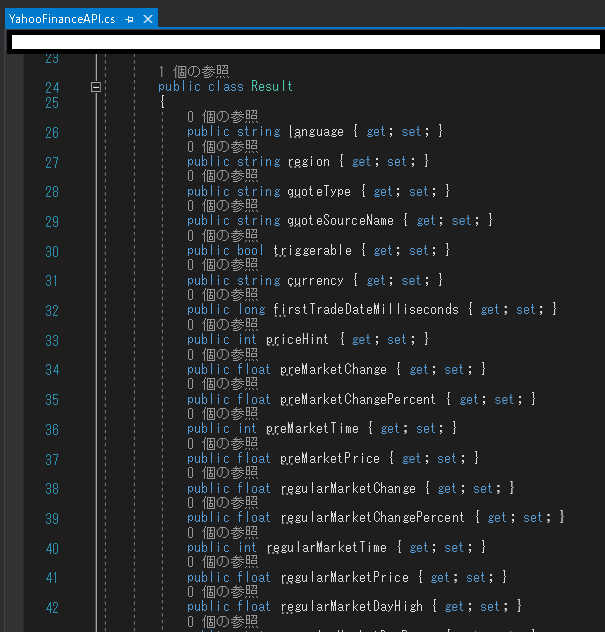
Yahoo Finance API の受信結果をデータクラスに変換する処理はこんな感じ。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
using System; using Newtonsoft.Json; using RestSharp; var client = new RestClient("https://stock-data-yahoo-finance-alternative.p.rapidapi.com/v6/finance/quote?symbols=AAPL%2CMSFT&lang=en®ion=US"); var request = new RestRequest(Method.GET); request.AddHeader("x-rapidapi-key", "〇〇〇"); request.AddHeader("x-rapidapi-host", "□□□"); var response = client.Execute<YahooFinanceApiResponse>(request); var yahooFinanceApiResponse = JsonConvert.DeserializeObject<YahooFinanceApiResponse>(response.Content); |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
public class YahooFinanceApiResponse { public Quoteresponse quoteResponse { get; set; } } public class Quoteresponse { public Result[] result { get; set; } public object error { get; set; } } public class Result { public string language { get; set; } public string region { get; set; } public string quoteType { get; set; } public string quoteSourceName { get; set; } public bool triggerable { get; set; } public string currency { get; set; } public long firstTradeDateMilliseconds { get; set; } public int priceHint { get; set; } public float preMarketChange { get; set; } public float preMarketChangePercent { get; set; } public int preMarketTime { get; set; } public float preMarketPrice { get; set; } public float regularMarketChange { get; set; } public float regularMarketChangePercent { get; set; } public int regularMarketTime { get; set; } public float regularMarketPrice { get; set; } public float regularMarketDayHigh { get; set; } public string regularMarketDayRange { get; set; } public float regularMarketDayLow { get; set; } public int regularMarketVolume { get; set; } public float regularMarketPreviousClose { get; set; } public float bid { get; set; } public float ask { get; set; } public int bidSize { get; set; } public int askSize { get; set; } public string fullExchangeName { get; set; } public string financialCurrency { get; set; } public float regularMarketOpen { get; set; } public int averageDailyVolume3Month { get; set; } public int averageDailyVolume10Day { get; set; } public float fiftyTwoWeekLowChange { get; set; } public float fiftyTwoWeekLowChangePercent { get; set; } public string fiftyTwoWeekRange { get; set; } public float fiftyTwoWeekHighChange { get; set; } public float fiftyTwoWeekHighChangePercent { get; set; } public float fiftyTwoWeekLow { get; set; } public float fiftyTwoWeekHigh { get; set; } public int dividendDate { get; set; } public int earningsTimestamp { get; set; } public int earningsTimestampStart { get; set; } public int earningsTimestampEnd { get; set; } public float trailingAnnualDividendRate { get; set; } public float trailingPE { get; set; } public float trailingAnnualDividendYield { get; set; } public float epsTrailingTwelveMonths { get; set; } public float epsForward { get; set; } public float epsCurrentYear { get; set; } public float priceEpsCurrentYear { get; set; } public long sharesOutstanding { get; set; } public float bookValue { get; set; } public float fiftyDayAverage { get; set; } public float fiftyDayAverageChange { get; set; } public float fiftyDayAverageChangePercent { get; set; } public float twoHundredDayAverage { get; set; } public float twoHundredDayAverageChange { get; set; } public float twoHundredDayAverageChangePercent { get; set; } public long marketCap { get; set; } public float forwardPE { get; set; } public float priceToBook { get; set; } public int sourceInterval { get; set; } public int exchangeDataDelayedBy { get; set; } public string averageAnalystRating { get; set; } public bool tradeable { get; set; } public string exchange { get; set; } public string longName { get; set; } public string messageBoardId { get; set; } public string exchangeTimezoneName { get; set; } public string exchangeTimezoneShortName { get; set; } public int gmtOffSetMilliseconds { get; set; } public string market { get; set; } public bool esgPopulated { get; set; } public string shortName { get; set; } public string marketState { get; set; } public string displayName { get; set; } public string symbol { get; set; } } |
コメント