前回作成した .NET 8.0 + Windowsフォームアプリ + Dapper + SQLite で実装するCRUD処理 に、CsvHelper NuGetパッケージをインストールし、Dapperを通して CSVファイルを SQLiteデータベースへインポートする機能を追加しました。CSVファイル処理の主流は CsvHelper。
ソースコードは GitHub で公開しています。
データベース
テーブル定義は前回作成したテーブルをそのまま使用。
1 2 3 4 5 6 7 8 9 |
CREATE TABLE "政権" ( "Id" INTEGER, "Start" TEXT, "End" TEXT, "Name" TEXT, PRIMARY KEY("Id") ) |
CSVファイル
テーブル定義に一致する、インポート用に作成したCSVファイル。
1 2 3 4 5 6 7 8 9 10 11 12 13 |
Id,Start,End,Name 1,2006-09-26,2007-09-26,安倍晋三 (第1期) 2,2007-09-26,2008-09-24,福田康夫 3,2008-09-24,2009-09-16,麻生太郎 4,2009-09-16,2010-06-08,鳩山由紀夫 5,2010-06-08,2011-09-02,菅直人 6,2011-09-02,2012-12-26,野田佳彦 7,2012-12-26,2020-09-16,安倍晋三 (第2期) 8,2020-09-16,2021-10-04,菅義偉 9,2021-10-04,2023-09-30,岸田文雄 10,2023-10-01,,石破茂 |
ソースコード構成
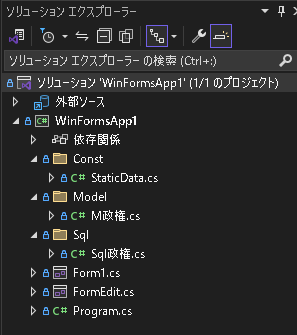
NuGet パッケージ インストール
CsvHelperパッケージをインストール
使用したバージョンは 2024/11/3時点で最新の v33.0.1。
1 2 3 |
> Install-Package CsvHelper |
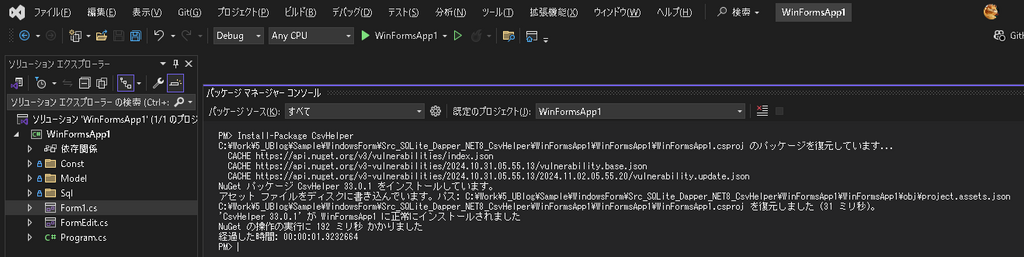
ソースコード変更内容を解説
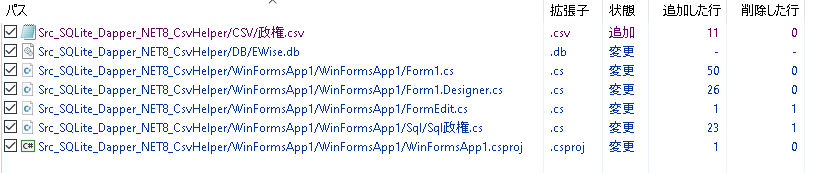
/CSV/政権.csv
インポート用に作成したCSVファイル。
/DB/EWise.db
前回、DB Browser for SQLiteで作成した SQLiteのデータベースファイルを流用。
/WinFormsApp1/WinFormsApp1/Form1.cs
CsvHelperの usingを追加。
「CSVファイルをインポート」ボタンの Clickイベントハンドラを追加。
「全データ削除」ボタンの Clickイベントハンドラを追加。
LoadCsv()メソッドで CsvHelperを使い CSVファイルをインポートし、M政権モデルクラスの Listへ変換している。CsvHelperを使用すると読み込んだファイルのデータをモデルクラスに変換する処理がシンプルになる。
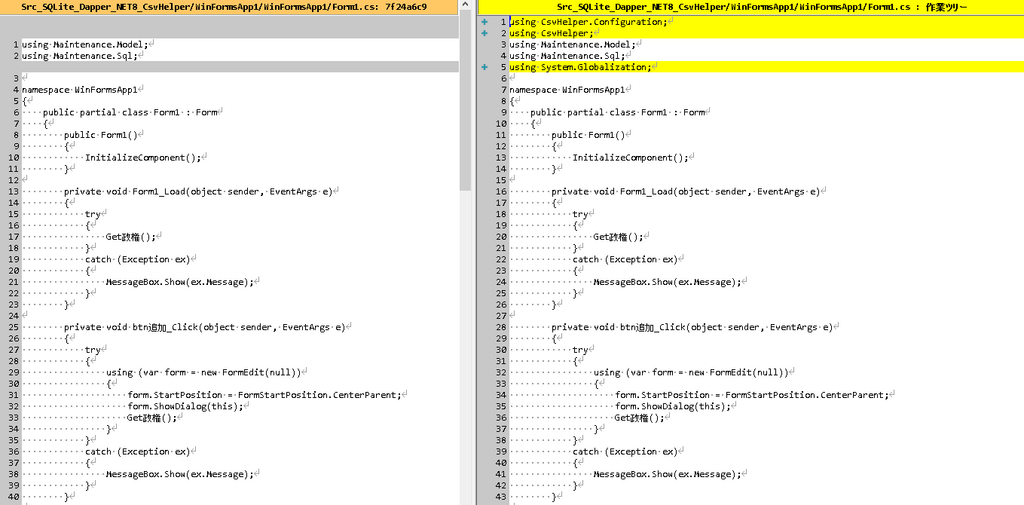
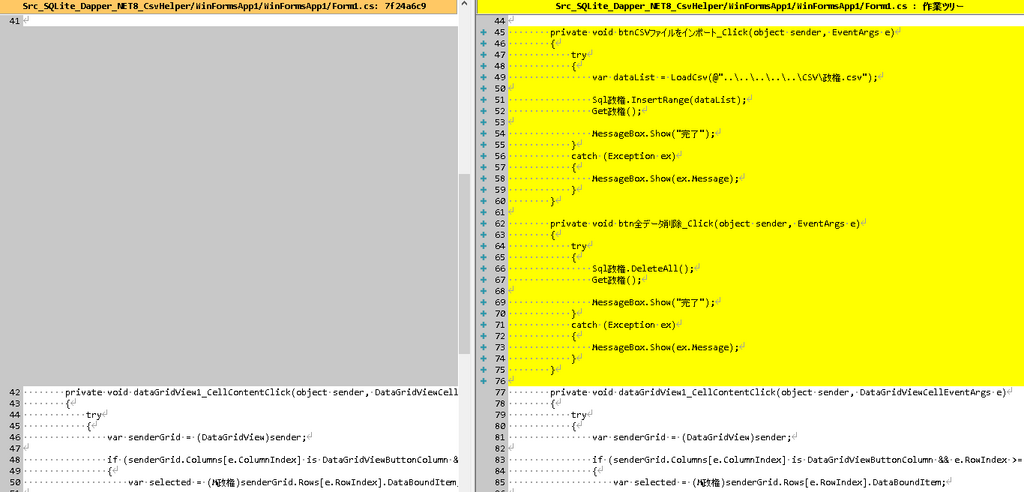
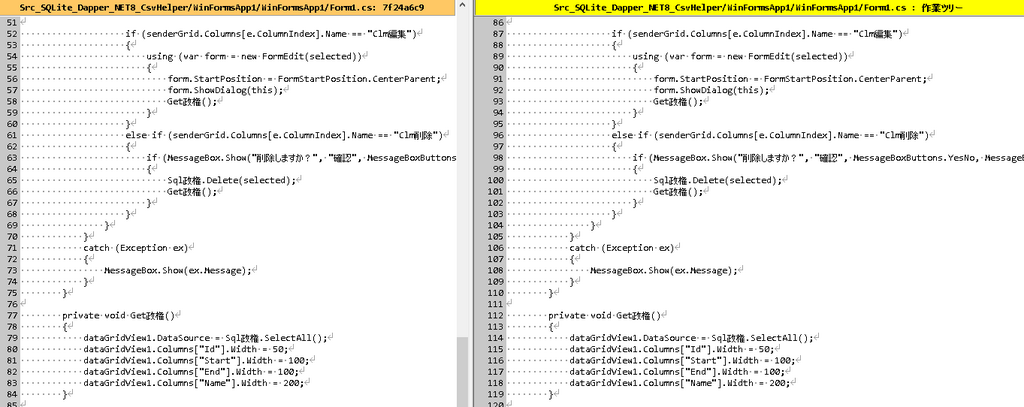
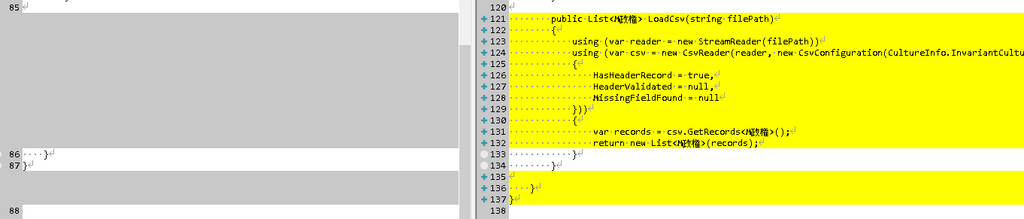
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 |
using CsvHelper.Configuration; using CsvHelper; using Maintenance.Model; using Maintenance.Sql; using System.Globalization; namespace WinFormsApp1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { try { Get政権(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btn追加_Click(object sender, EventArgs e) { try { using (var form = new FormEdit(null)) { form.StartPosition = FormStartPosition.CenterParent; form.ShowDialog(this); Get政権(); } } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btnCSVファイルをインポート_Click(object sender, EventArgs e) { try { var dataList = LoadCsv(@"..\..\..\..\..\CSV\政権.csv"); Sql政権.InsertRange(dataList); Get政権(); MessageBox.Show("完了"); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btn全データ削除_Click(object sender, EventArgs e) { try { Sql政権.DeleteAll(); Get政権(); MessageBox.Show("完了"); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e) { try { var senderGrid = (DataGridView)sender; if (senderGrid.Columns[e.ColumnIndex] is DataGridViewButtonColumn && e.RowIndex >= 0) { var selected = (M政権)senderGrid.Rows[e.RowIndex].DataBoundItem; if (senderGrid.Columns[e.ColumnIndex].Name == "Clm編集") { using (var form = new FormEdit(selected)) { form.StartPosition = FormStartPosition.CenterParent; form.ShowDialog(this); Get政権(); } } else if (senderGrid.Columns[e.ColumnIndex].Name == "Clm削除") { if (MessageBox.Show("削除しますか?", "確認", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes) { Sql政権.Delete(selected); Get政権(); } } } } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void Get政権() { dataGridView1.DataSource = Sql政権.SelectAll(); dataGridView1.Columns["Id"].Width = 50; dataGridView1.Columns["Start"].Width = 100; dataGridView1.Columns["End"].Width = 100; dataGridView1.Columns["Name"].Width = 200; } public List<M政権> LoadCsv(string filePath) { using (var reader = new StreamReader(filePath)) using (var csv = new CsvReader(reader, new CsvConfiguration(CultureInfo.InvariantCulture) { HasHeaderRecord = true, HeaderValidated = null, MissingFieldFound = null })) { var records = csv.GetRecords<M政権>(); return new List<M政権>(records); } } } } |
/WinFormsApp1/WinFormsApp1/Form1.Designer.cs
画面レイアウトに「CSVファイルをインポート」「全データ削除」ボタンを追加。
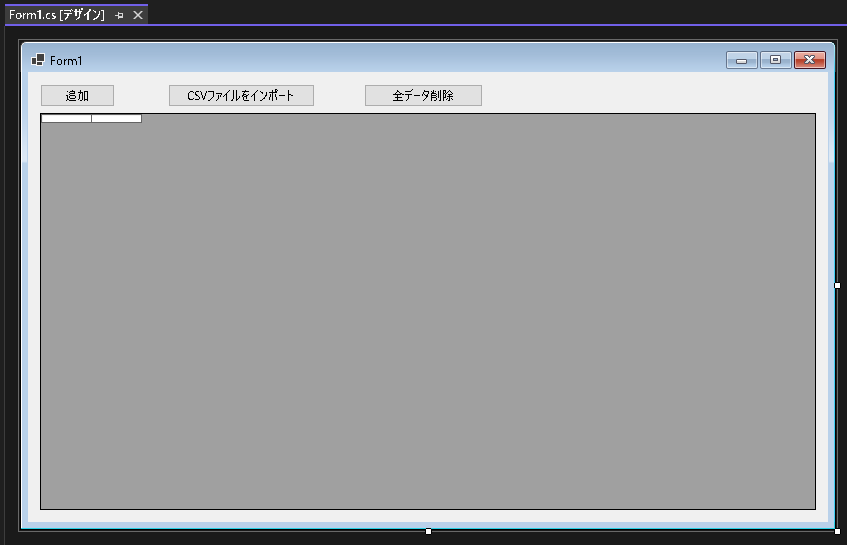
/WinFormsApp1/WinFormsApp1/FormEdit.cs
メソッド名を Add()から Insert()へ変更したのみ。
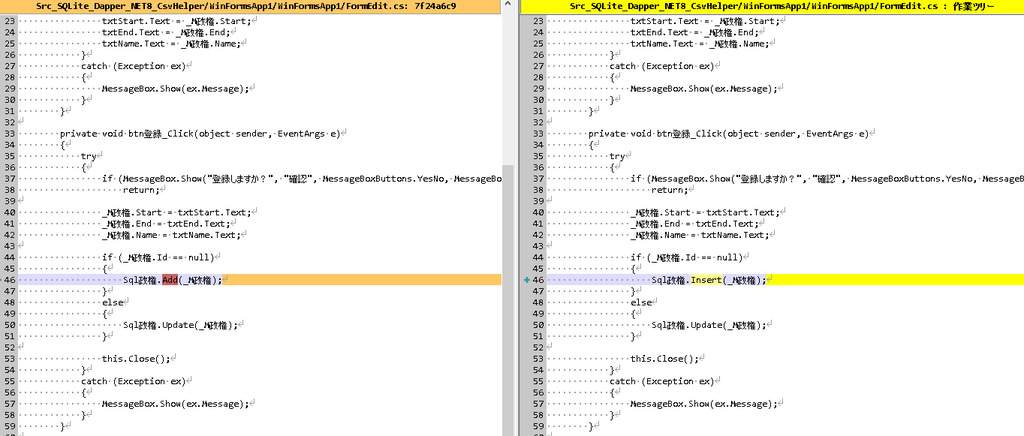
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
using Maintenance.Model; using Maintenance.Sql; using System.Xml.Linq; namespace WinFormsApp1 { public partial class FormEdit : Form { private M政権 _M政権; public FormEdit(M政権 m政権) { InitializeComponent(); _M政権 = m政権 == null ? new M政権() : m政権; } private void FormEdit_Load(object sender, EventArgs e) { try { txtId.Text = _M政権.Id.ToString(); txtStart.Text = _M政権.Start; txtEnd.Text = _M政権.End; txtName.Text = _M政権.Name; } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btn登録_Click(object sender, EventArgs e) { try { if (MessageBox.Show("登録しますか?", "確認", MessageBoxButtons.YesNo, MessageBoxIcon.Question) != DialogResult.Yes) return; _M政権.Start = txtStart.Text; _M政権.End = txtEnd.Text; _M政権.Name = txtName.Text; if (_M政権.Id == null) { Sql政権.Insert(_M政権); } else { Sql政権.Update(_M政権); } this.Close(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btnキャンセル_Click(object sender, EventArgs e) { try { this.Close(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } } } |
/WinFormsApp1/WinFormsApp1/Sql/Sql政権.cs
メソッド名を Add()から Insert()へ変更。
読み込んだ CSVファイルのデータを SQLiteへ登録する InsertRange()メソッドを追加。
Dapperを使うことで Listデータをループせずに一括Insertしている。
便宜的にテーブルデータを全件削除する DeleteAll()メソッドを追加。
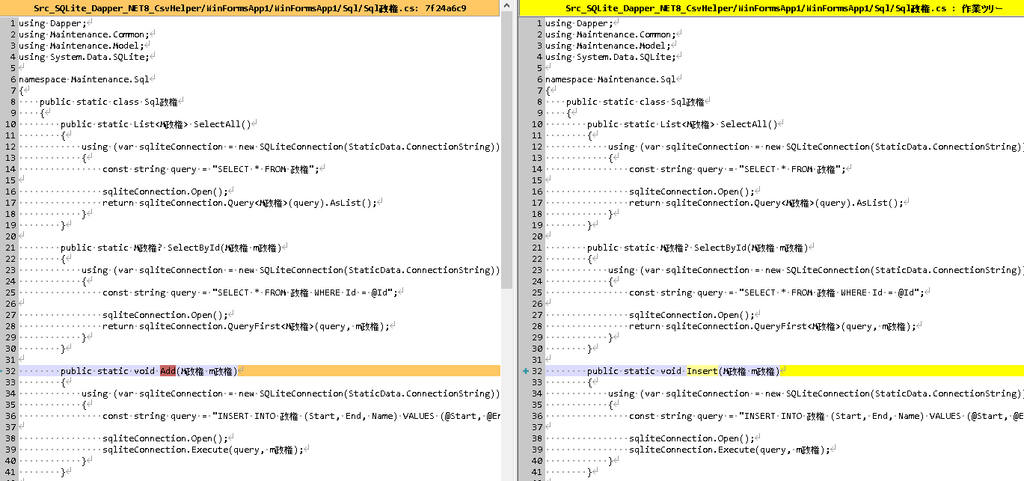
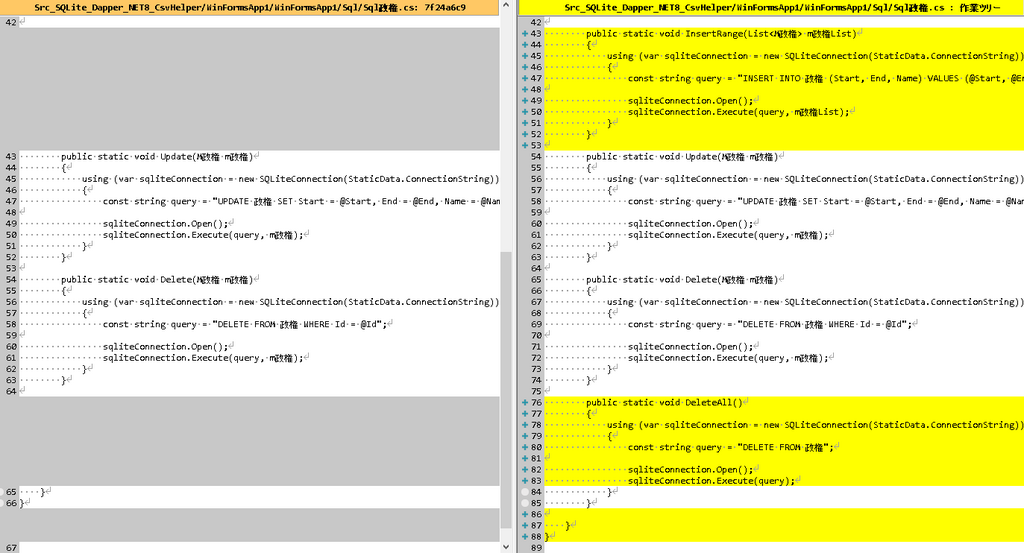
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
using Dapper; using Maintenance.Common; using Maintenance.Model; using System.Data.SQLite; namespace Maintenance.Sql { public static class Sql政権 { public static List<M政権> SelectAll() { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "SELECT * FROM 政権"; sqliteConnection.Open(); return sqliteConnection.Query<M政権>(query).AsList(); } } public static M政権? SelectById(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "SELECT * FROM 政権 WHERE Id = @Id"; sqliteConnection.Open(); return sqliteConnection.QueryFirst<M政権>(query, m政権); } } public static void Insert(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "INSERT INTO 政権 (Start, End, Name) VALUES (@Start, @End, @Name)"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権); } } public static void InsertRange(List<M政権> m政権List) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "INSERT INTO 政権 (Start, End, Name) VALUES (@Start, @End, @Name)"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権List); } } public static void Update(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "UPDATE 政権 SET Start = @Start, End = @End, Name = @Name WHERE Id = @Id"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権); } } public static void Delete(M政権 m政権) { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "DELETE FROM 政権 WHERE Id = @Id"; sqliteConnection.Open(); sqliteConnection.Execute(query, m政権); } } public static void DeleteAll() { using (var sqliteConnection = new SQLiteConnection(StaticData.ConnectionString)) { const string query = "DELETE FROM 政権"; sqliteConnection.Open(); sqliteConnection.Execute(query); } } } } |
/WinFormsApp1/WinFormsApp1/WinFormsApp1.csproj
インストールした CsvHelperが追加されている。
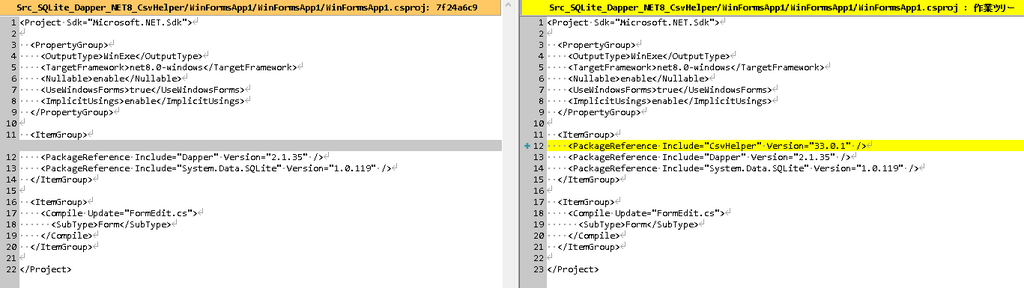
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <OutputType>WinExe</OutputType> <TargetFramework>net8.0-windows</TargetFramework> <Nullable>enable</Nullable> <UseWindowsForms>true</UseWindowsForms> <ImplicitUsings>enable</ImplicitUsings> </PropertyGroup> <ItemGroup> <PackageReference Include="CsvHelper" Version="33.0.1" /> <PackageReference Include="Dapper" Version="2.1.35" /> <PackageReference Include="System.Data.SQLite" Version="1.0.119" /> </ItemGroup> <ItemGroup> <Compile Update="FormEdit.cs"> <SubType>Form</SubType> </Compile> </ItemGroup> </Project> |
コメント