.NET 6.0 の CoreWCFサーバ側で、WCF通信時の HttpRequest Header情報を取得するサンプルを作成しました。
ソースコードはGitHubで公開しています。
収集できたHeader情報。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Host : localhost:7086 Accept-Encoding : gzip, deflate Cache-Control : no-cache, max-age=0 Content-Type : text/xml; charset=utf-8 Content-Length : 161 SOAPAction : "http://tempuri.org/IService/GetData" Path : /Service.svc Method : POST Host : localhost:7086 Protocol : HTTP/1.1 ContentType : text/xml; charset=utf-8 ContentLength : 161 IsHttps : True |
ソースコード構成
.NET6.0で実装する Core WCF 開発手順 で作成したソースコードに、下記の修正を加えました。

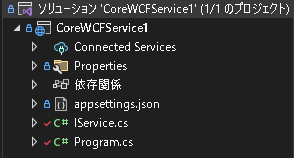
ソースコード変更内容を解説
/Program.cs
Serviceクラスで DI [Injected]を使えるように、Serviceクラスを AddTransientする処理を追加。
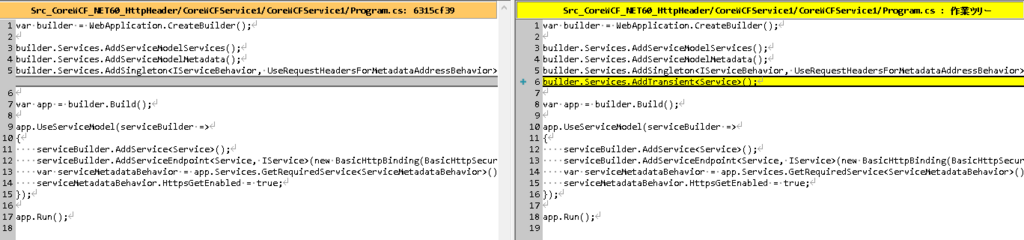
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
var builder = WebApplication.CreateBuilder(); builder.Services.AddServiceModelServices(); builder.Services.AddServiceModelMetadata(); builder.Services.AddSingleton<IServiceBehavior, UseRequestHeadersForMetadataAddressBehavior>(); builder.Services.AddTransient<Service>(); var app = builder.Build(); app.UseServiceModel(serviceBuilder => { serviceBuilder.AddService<Service>(); serviceBuilder.AddServiceEndpoint<Service, IService>(new BasicHttpBinding(BasicHttpSecurityMode.Transport), "/Service.svc"); var serviceMetadataBehavior = app.Services.GetRequiredService<ServiceMetadataBehavior>(); serviceMetadataBehavior.HttpsGetEnabled = true; }); app.Run(); |
/IService.cs
GetDataメソッドに HttpResponse・HttpRequest DIパラメータを追加。
HttpRequestの主要なキーと値を StringBuilder変数に出力し確認出来る処理を追加。
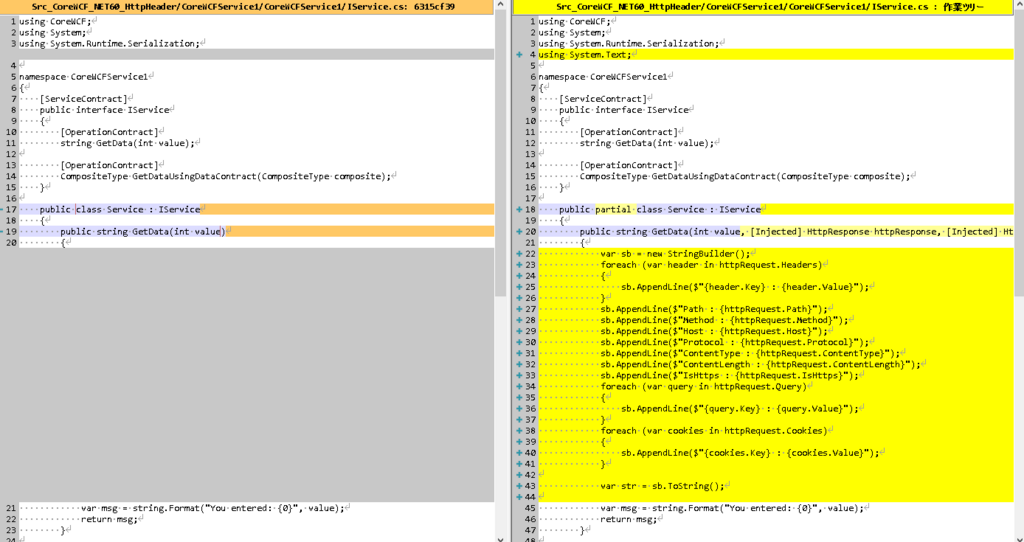
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 |
using CoreWCF; using System; using System.Runtime.Serialization; using System.Text; namespace CoreWCFService1 { [ServiceContract] public interface IService { [OperationContract] string GetData(int value); [OperationContract] CompositeType GetDataUsingDataContract(CompositeType composite); } public partial class Service : IService { public string GetData(int value, [Injected] HttpResponse httpResponse, [Injected] HttpRequest httpRequest) { var sb = new StringBuilder(); foreach (var header in httpRequest.Headers) { sb.AppendLine($"{header.Key} : {header.Value}"); } sb.AppendLine($"Path : {httpRequest.Path}"); sb.AppendLine($"Method : {httpRequest.Method}"); sb.AppendLine($"Host : {httpRequest.Host}"); sb.AppendLine($"Protocol : {httpRequest.Protocol}"); sb.AppendLine($"ContentType : {httpRequest.ContentType}"); sb.AppendLine($"ContentLength : {httpRequest.ContentLength}"); sb.AppendLine($"IsHttps : {httpRequest.IsHttps}"); foreach (var query in httpRequest.Query) { sb.AppendLine($"{query.Key} : {query.Value}"); } foreach (var cookies in httpRequest.Cookies) { sb.AppendLine($"{cookies.Key} : {cookies.Value}"); } var str = sb.ToString(); var msg = string.Format("You entered: {0}", value); return msg; } public CompositeType GetDataUsingDataContract(CompositeType composite) { if (composite == null) { throw new ArgumentNullException("composite"); } if (composite.BoolValue) { composite.StringValue += "Suffix"; } return composite; } } // Use a data contract as illustrated in the sample below to add composite types to service operations. [DataContract] public class CompositeType { bool boolValue = true; string stringValue = "Hello "; [DataMember] public bool BoolValue { get { return boolValue; } set { boolValue = value; } } [DataMember] public string StringValue { get { return stringValue; } set { stringValue = value; } } } } |
コメント