Angularで特定のコンポーネントをデフォルト表示する手順 で作成した Angularプロジェクトに、AngularでWebAPIからテキストデータを取得して画面に表示する機能を追加しました。
WebAPIは ASP.NET Core Web API で実装しています。
ソースコードは GitHub で公開しています。
- WebAPI側実装
- Angular側実装
- サービスコンポーネント作成
- 追加変更したソースコード解説
- Src7_Add_InputText_WebAPI/angular/src/app/app.config.ts
- Src7_Add_InputText_WebAPI/angular/src/app/profile/profile.component.html
- Src7_Add_InputText_WebAPI/angular/src/app/profile/profile.component.ts
- Src7_Add_InputText_WebAPI/angular/src/app/services/profile.service.spec.ts
- Src7_Add_InputText_WebAPI/angular/src/app/services/profile.service.ts
- 実装した環境
WebAPI側実装
WebAPIプロジェクト作成
「ASP.NET Core Web API」を選択し「次へ」をクリック。
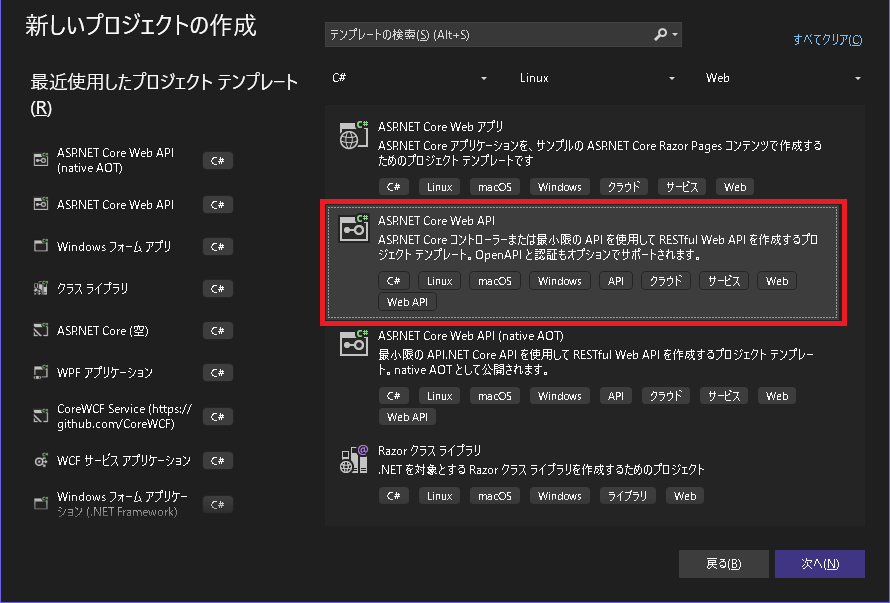
任意の値を入力し「次へ」をクリック。
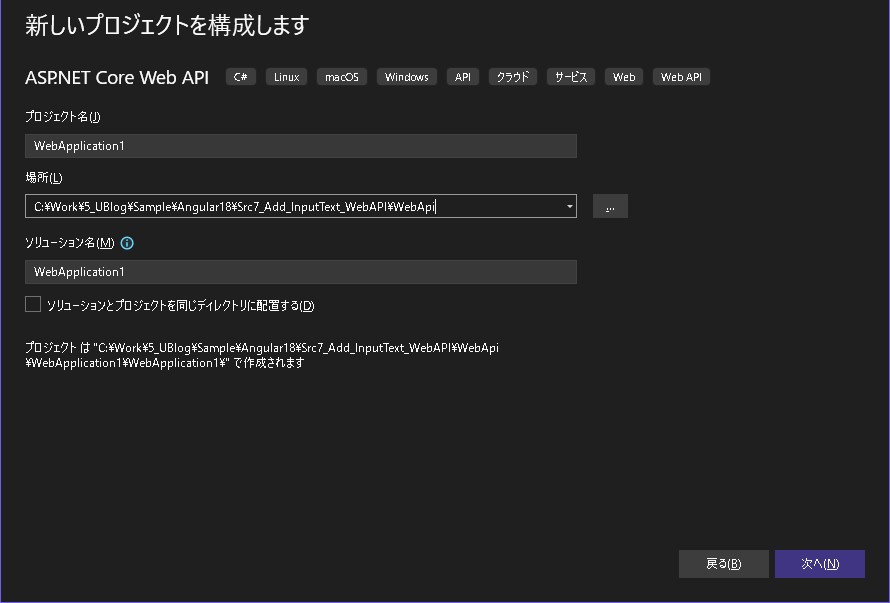
任意の値を入力し「作成」をクリック。
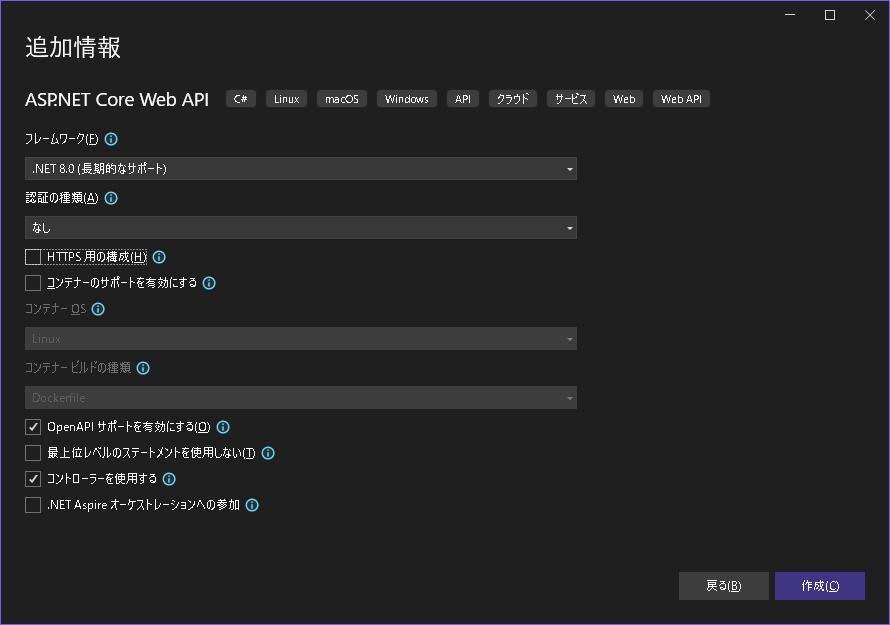
追加変更したソースコード解説
「ASP.NET Core Web API」プロジェクトを新規作成した時点から、追加変更したソースコードを解説。

Src7_Add_InputText_WebAPI/WebApi/WebApplication1/WebApplication1/Controllers/ProfileController.cs
Angularが接続するWebAPIのコントローラーを追加。
テキストデータをGETプロトコルで返す GetString()メソッドを追加。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; namespace WebApplication1.Controllers { [Route("api/[controller]")] [ApiController] public class ProfileController : ControllerBase { [HttpGet("GetString")] public async Task<ActionResult> GetString() { return Ok("test string."); //return BadRequest("Error occurred"); } } } |
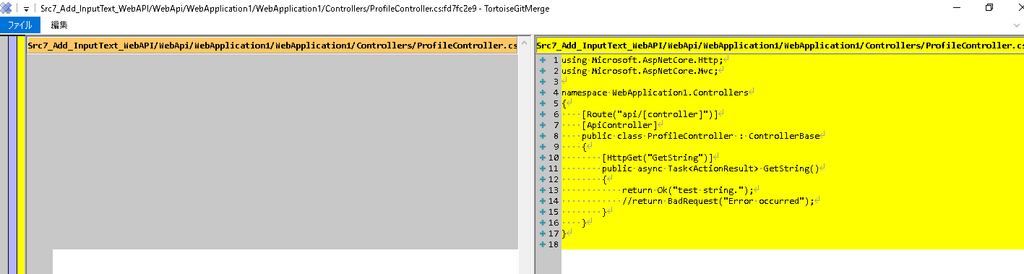
Src7_Add_InputText_WebAPI/WebApi/WebApplication1/WebApplication1/Program.cs
UseCors()を追加し開発環境ではCORSを全て許可。
1 2 3 |
app.UseCors(policy => policy.AllowAnyOrigin().AllowAnyHeader().AllowAnyMethod()); |
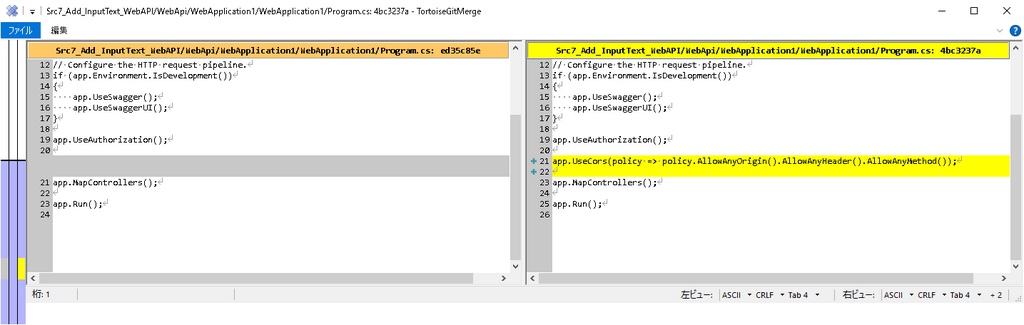
CORSを許可していないと、デバッグ実行したWebAPIを Angularから呼び出す際に下記エラーが発生する。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
Access to fetch at 'http://localhost:5068/api/Profile' from origin 'http://localhost:4200' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled. Failed to load resource: net::ERR_FAILED profile.component.ts:28 Error: HttpErrorResponse profile.component.ts:28 Error: HttpErrorResponse error @ profile.component.ts:28 rejected @ chunk-IKJ4S2J2.js?v=991de893:43 Promise.then step @ chunk-IKJ4S2J2.js?v=991de893:48 (anonymous) @ chunk-IKJ4S2J2.js?v=991de893:49 __async @ chunk-IKJ4S2J2.js?v=991de893:33 getString @ profile.component.ts:23 ngOnInit @ profile.component.ts:19 |
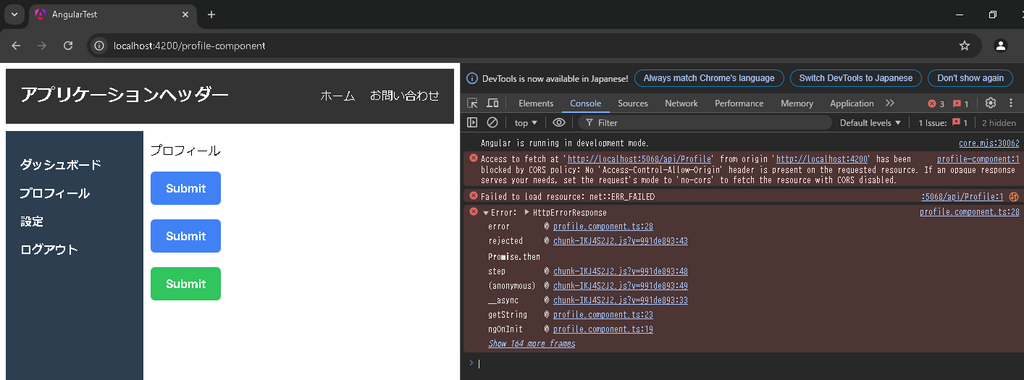
Angular側実装
サービスコンポーネント作成
WebAPIを処理する用にサービスコンポーネントを追加。
1 2 3 |
> ng generate service services/profile |
作成された。
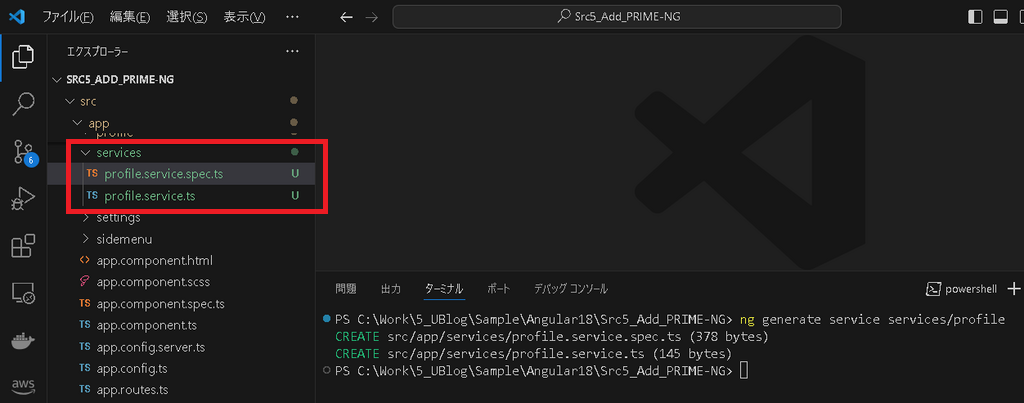
追加変更したソースコード解説

Src7_Add_InputText_WebAPI/angular/src/app/app.config.ts
http処理に必要なライブラリをインポート。
1 2 3 |
import { provideHttpClient, withFetch } from '@angular/common/http'; |
1 2 3 |
provideHttpClient(withFetch() |
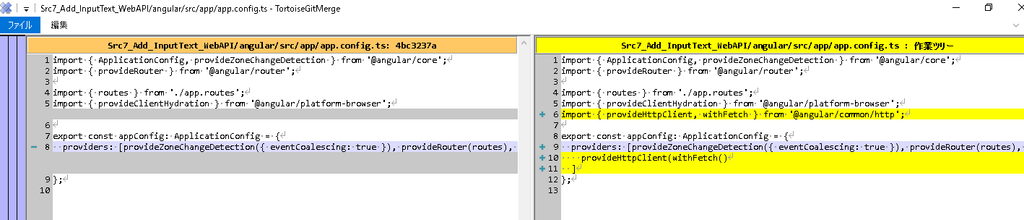
Src7_Add_InputText_WebAPI/angular/src/app/profile/profile.component.html
profile.component.tsに追加した resString変数を画面に表示する処理を追加。
1 2 3 |
<p>{{ this.resString }}</p> |

Src7_Add_InputText_WebAPI/angular/src/app/profile/profile.component.ts
「ng generate service services/profile」コマンドで作成したサービスを画面にインポート。
1 2 3 |
import { ProfileService } from '../services/profile.service'; |
サービスから取得したテキストデータを格納し画面に表示するための resString変数を追加。
画面表示初期イベント(ngOnInit)で、サービスの getString()メソッドを非同期(subscribe)で実行し、結果を resString変数に格納する。
1 2 3 4 5 6 7 8 9 10 11 |
resString: string = ''; constructor(private profileService: ProfileService) {} ngOnInit(): void { this.profileService.getString().subscribe(res => { this.resString = res; }); } |
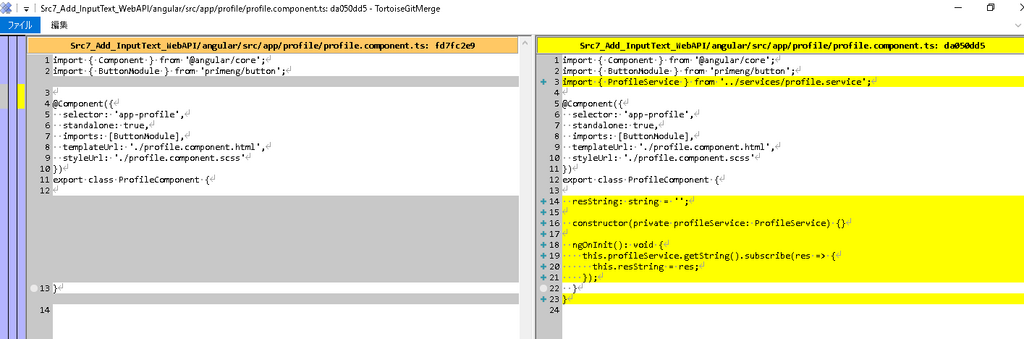
Src7_Add_InputText_WebAPI/angular/src/app/services/profile.service.spec.ts
※「ng generate service services/profile」コマンドで作成した時点から変更ないので省略。
Src7_Add_InputText_WebAPI/angular/src/app/services/profile.service.ts
「ng generate service services/profile」コマンドで作成したサービスに、WebAPIからテキストデータを取得する getString()メソッドを追加。
getString()メソッドは rxjsを利用し、WebAPIから取得したテキストデータを非同期(Observables)で返している。
WebAPIとの通信で何らかのエラーが発生した場合のエラー処理は、rxjsの catchErrorでハンドリングしている。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
import { HttpClient } from '@angular/common/http'; import { Injectable } from '@angular/core'; import { catchError, of } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class ProfileService { private apiUrl = 'http://localhost:5068/api/Profile/GetString'; constructor(private http: HttpClient) { } getString() { return this.http.get<string>(this.apiUrl, { responseType: 'text' as 'json' }).pipe( catchError(err => { console.error('Error:', err); return of(''); }) ); } } |
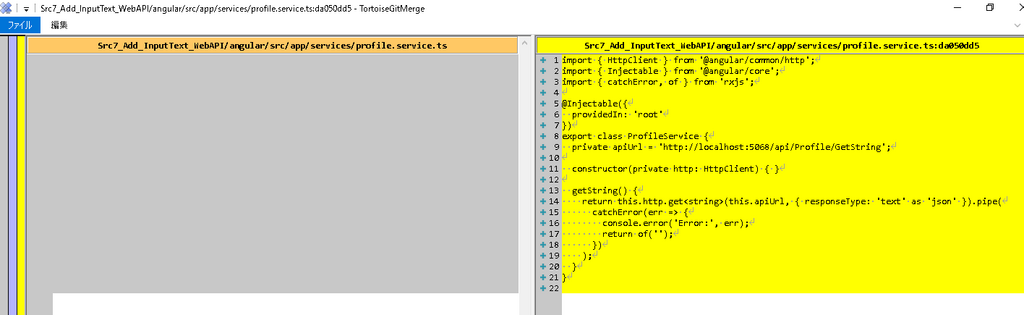
実装した環境
Angular側
Angular: 18.2.3
Node.js: 22.8.0
PrimeNG: 17.18.10
Visual Studio Code: 1.93.1
WebAPI側
Visual Studio 2022: 17.11.4
コメント